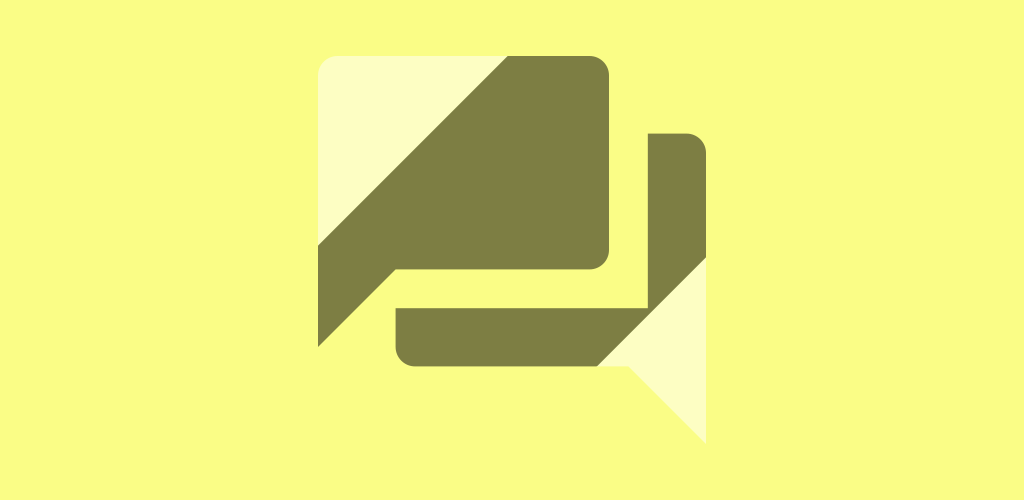
- 23 April, 2025
- 4 Min read
Interview | Synechron
The interview process for a Node.js Developer at Synechron, with 5 years of experience.
- Akshahy Kumar
- Interview Web Patterns
- Synechron
- Hyderabad, Telangana, India
Organization
At Synechron, we believe in the power of digital to transform businesses for the better. Our global consulting firm combines creativity and innovative technology to deliver industry-leading digital solutions. Synechron’s progressive technologies and optimization strategies span end-to-end Artificial Intelligence, Consulting, Digital, Cloud & DevOps, Data, and Software Engineering, servicing an array of noteworthy financial services and technology firms. Through research and development initiatives in our FinLabs we develop solutions for modernization, from Artificial Intelligence and Blockchain to Data Science models, Digital Underwriting, mobile-first applications and more.
Over the last 20+ years, our company has been honored with multiple employer awards, recognizing our commitment to our talented teams. With top clients to boast about, Synechron has a global workforce of 14,000+, and has 55 offices in 20 countries within key global markets. For more information on the company, please visit our website: www.synechron.com.
Company
Founded: 2001
Size: 10,001+ employees
Headquarter: New York, NY, US
Specialties: Digital, Technology, Data, Systems Integration, Payments, Engineering, ESG, Sustainable Finance, Innovation, Digital Transformation, Cloud & DevOps, Consulting, and Artificial Intelligence
ToC
Background
I registered on Naukri.com
and uploaded my resume there and at around 11:00 am, I got a call from the recruiter. She said she is a third-party recruiter for Synechron, and it means that the Synechron company has given them a contract for recruiting Software Engineers.
Wikipedia
Naukri.com is an Indian employment website which operates in India and the Middle East. It was founded in March 1997 bySanjeev Bikhchandani
as a division of Info Edge. It is the largest employment website in India.
Screening
After getting the call from the recruiter, they asked me some questions related to my work experience, package, and availability.
Discussion
- How many years of IT industry experience do you have?
- How many years of relevant experience do you have in
Node.js
? - Do you have any team lead experience?
- If yes, then how many team members have you handled?
- What is your notice period and how soon can you join?
- What is your current salary package and what are you expecting?
- Are you comfortable with working from the office (5 days a week)?
I was told that they would submit the details to the company and if Synechron shortlists me, then I will get the further details via email which I have to acknowledge to start the interview process.
After some days, that third-party recruiter sent me the interview invite over Teams via email which happened virtually.
Interview
The interview started with a brief introduction followed by various questions related to JavaScript.
JavaScript
Tactic
JavaScript, often abbreviated as JS
, is a programming language and core technology of the World Wide Web, alongside HTML and CSS. Ninety-nine percent of websites use JavaScript on the client side for webpage behavior.
Web browsers have a dedicated JavaScript engine that executes the client code. These engines are also utilized in some servers and a variety of apps. The most popular runtime system for non-browser usage is Node.js.
JavaScript is a high-level, often just-in-time–compiled language that conforms to the ECMAScript
standard. It has dynamic typing, prototype-based object-orientation, and first-class functions. It is multi-paradigm, supporting event-driven, functional, and imperative programming styles. It has application programming interfaces (APIs) for working with text, dates, regular expressions, standard data structures, and the Document Object Model (DOM).
Although Java and JavaScript are similar in name and syntax, the two languages are distinct and differ greatly in design.
The ECMAScript standard does not include any input/output (I/O), such as networking, storage, or graphics facilities. In practice, the web browser or other runtime system provides JavaScript APIs for I/O.
TypeScript
TypeScript (abbreviated as TS
) is a free and open-source high-level programming language developed by Microsoft
that adds static typing
with optional type annotations to JavaScript. It is designed for the development of large applications and transpiles to JavaScript.
JavaScript / TypeScript
- What is
hoisting
in JavaScript? - What are the
generics
in TypeScript? - What is
mocking
in Testing? - What are the disadvantages of
closures
?
Output
setTimeout(() => {
console.log("I m set Timeout")
}, 0);
setImmediate(() => {
console.log('I m imm')
});
process.nextTick(()=>{
console.log('I m tick');
});
// Identify the output sequence.
for(var i = 0; i < 3; i++){
const log = () => {
console.log(i);
}
setTimeout(log, 100);
}
Complexity
function doSomething(m, n) {
for(let i = 0; i <= m; i++) {
...
}
for(let i = 0; i <= n; i++) {
...
}
}
// Time Complexity = ?
// Space Complexity = ?
Node.js
Tactic
Node.js is a cross-platform, open-source JavaScript runtime environment that can run on Windows, Linux, Unix, macOS, and more. Node.js runs on the Chrome’s V8 JavaScript engine, and executes JavaScript code outside a web browser. It is a free, open-source, cross-platform JavaScript runtime environment licensed under MIT License
that lets developers create servers, web apps, command line tools and scripts.
Node.js lets developers use JavaScript to write command line tools and for server-side scripting. The ability to run JavaScript code on the server is often used to generate dynamic web page content before the page is sent to the user’s web browser. Consequently, Node.js represents a “JavaScript everywhere” paradigm, unifying web-application development around a single programming language, as opposed to using different languages for the server- versus client-side programming.
Node / JS
- How to set
environment variables
in Node.js? - What are the
phases
of the Event Loop in Node.js? - Which has more
priority
in whether Microtask Queue or Macrotask Queue? - What is Idempotency in APIs?
Security
- If you encrypt the password in the API and the
admin
got access to the secret key of the password, how would you save the password from illegal access if someone got the secret key? - What is
hashing
in Cryptography?
Patterns
A full-stack developer is also responsible for creating the architecture and connecting things together.
Technique
In software engineering, a software design pattern or design pattern
is a general, reusable solution to a commonly occurring problem in many contexts in software design. A design pattern is not a rigid structure to be transplanted directly into source code. Rather, it is a description or a template
for solving a particular type of problem that can be deployed in many different situations. Design patterns can be viewed as formalized best practices that the programmer may use to solve common problems when designing a software application or system.
Object-oriented design patterns typically show relationships and interactions between classes or objects, without specifying the final application classes or objects that are involved. Patterns that imply mutable state may be unsuited for functional programming languages. Some patterns can be rendered unnecessary in languages that have built-in support for solving the problem they are trying to solve, and object-oriented patterns are not necessarily suitable for non-object-oriented languages.
Design patterns may be viewed as a structured approach to computer programming intermediate between the levels of a programming paradigm and a concrete algorithm.
Design Patterns
- What are Solid Principles?
- What are Design Patterns and their use?
- What is
Factory Design Pattern
? Name some others as well. - What is
API
Gateway? - What is API Gateway Integrations like
3scale
? - What is Event Driven Architecture like
Kafka
?
Microservices
- What are
Observer
,SAGA
,Circuit Breaker
,Service Discovery
, etc.?
Database
- What is ACID?
- What is
Redis
? - What is Sharding?
- What is Left Join?
- What is
isolation
in Database? - What is Normalization and Denormalization?
DevOps
To deploy end-to-end products, a developer should know the basics of developer operations as well.
Tactic
DevOps is the integration
and automation
of the software development and information technology operations. DevOps encompasses necessary tasks of software development and can lead to shortening development time and improving the development life cycle.
According to Neal Ford, DevOps, particularly through continuous delivery, employs the “Bring the pain forward” principle, tackling tough tasks early, fostering automation and swift issue detection. Software programmers and architects should use fitness functions to keep their software in check.
Although debated, DevOps is characterized by key principles: shared ownership, workflow automation, and rapid feedback.
From an academic perspective, Len Bass, Ingo Weber, and Liming Zhu—three computer science researchers from the CSIRO and the Software Engineering Institute—suggested defining DevOps as “a set of practices intended to reduce the time between committing a change to a system and the change being placed into normal production, while ensuring high quality”. However, the term is used in multiple contexts.
At its most successful, DevOps is a combination of specific practices, culture change, and tools.
Containerization
In software engineering, containerization is operating-system–level virtualization or application-level virtualization over multiple network resources so that software applications can run in isolated user spaces called containers
in any cloud or non-cloud environment, regardless of type or vendor. The term “container” is overloaded, and it is important to ensure that the intended definition aligns with the audience’s understanding.
Container
orchestration
or container management is mostly used in the context of application containers. Implementations providing such orchestration includeKubernetes
andDocker
swarm.
DevOps
- How to do
CI/CD
? - Difference between
Vertical
andHorizontal
Scaling? - What are the
techniques
, we can use in Horizontal Scaling?
What are Containerization,
Docker
andKubernetes
?
We are done for now, the interviewer said and asked me if I had any questions.
Conclusion
This interview lasted over an hour, and it happened at 9:00 pm. Suddenly, I got a call from the recruiter at 8:30 pm, and she said that the interviewer doesn’t have the time slots in the daytime, so they urgently wanted to take the interview tonight at 9:00 pm. I asked them to postpone it for another day, but they declined my request. So, I gave the interview with less energy because I was feeling lethargic at that time, as my biological clock is not set for this odd time.
I started giving the interview with the universal question, “Please tell me about yourself”, then they asked me a series of questions, which are mentioned above.
For most of the questions, I responded well and gave the answers correctly. Some of them were mentally intensive, and I couldn’t provide a better answer. In the end, they said we will get back to you, but they haven’t yet.
Takeaways
- Do
pranayam
to keep yourself mentally and physically fit. - Always be ready for the interview, not just during the day.