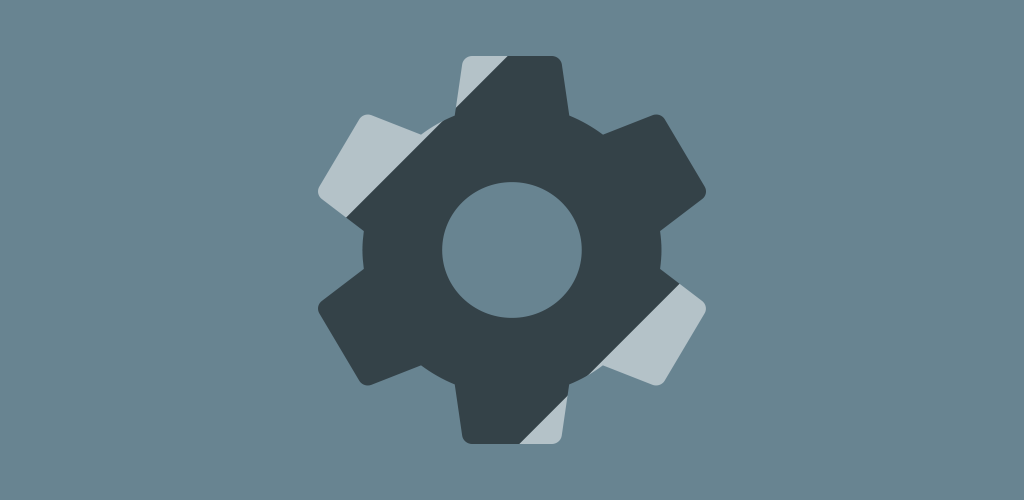
- 26 June, 2017
- 7 Min read
Dynamic Utils
Utility functions to perform dynamic operations on Android.
A collection of static methods to perform various operations including color, device, drawable, package and SDK on Android 2.3 (API 9) and above devices.
Background
I have several apps on Google Play and sometimes it is required to perform quick operations without leaving the context. So, I have developed this library to make a collection of such methods that can be used from anywhere within an Android app.
Usage
It is divided into different classes according to their category for easy understanding and usage. This library is fully commented so I am highlighting some of the features below.
Please follow and keep exploring the GitHub repository to find out more hidden features.
DynamicAnimUtils
Helper class to perform animation
related operations.
/** * Play an animator animation on a view. * * @param view The view to play the animation. * @param animator The animator to be applied on the view. */void playAnimation(@NonNull View view, @AnimatorRes int animator);
DynamicBitmapUtils
Helper class to perform bitmap
operations.
/** * Get bitmap from the supplied drawable. * * @param drawable The drawable to get the bitmap. * @param width The width in dip for the bitmap. * @param height The height in dip for the bitmap. * @param compress {@code true} to compress the bitmap. * @param quality The quality of the compressed bitmap. * * @return The bitmap from the supplied drawable. */@Nullable Bitmap getBitmapFromDrawable(@Nullable Drawable drawable, int width, int height, boolean compress, int quality);
/** * Creates a bitmap from the supplied view. * * @param view The view to get the bitmap. * @param width The width for the bitmap. * @param height The height for the bitmap. * * @return The bitmap from the supplied drawable. */@NonNull Bitmap createBitmapFromView(@NonNull View view, int width, int height);
DynamicColorUtils
Helper class to change colors
dynamically.
/** * Adjust alpha of a color according to the given parameter. * * @param color The color whose alpha to be adjusted. * @param factor Factor in float by which adjust the alpha. * * @return The color with adjusted alpha. */@ColorInt int adjustAlpha(@ColorInt int color, float factor);
/** * Calculate tint based on a given color for better readability. * * @param color The color whose tint to be calculated. * * @return The tint of the given color. */@ColorInt int getTintColor(@ColorInt int color);
DynamicDeviceUtils
Helper class to detect device
specific features like Telephony
, etc.
/** * Detects the telephony feature by using {@link PackageManager}. * * @param context The context to get the package manager. * * @return {@code true} if the device has telephony feature. * * @see PackageManager#hasSystemFeature(String) * @see PackageManager#FEATURE_TELEPHONY */boolean hasTelephony(@NonNull Context context);
DynamicDrawableUtils
Helper class to perform drawable
operations.
/** * Colorize and return the mutated drawable so that, all other references do not change. * * @param drawable The drawable to be colorized. * @param color The color to colorize the drawable. * @param wrap {@code true} to {@code wrap} the drawable so that it may be used for * tinting across the different API levels. * @param mode The porter duff mode. * * @return The drawable after applying the color filter. * * @see Drawable#setColorFilter(int, PorterDuff.Mode) * @see PorterDuff.Mode */@Nullable Drawable colorizeDrawable(@Nullable Drawable drawable, boolean wrap, @ColorInt int color, @Nullable PorterDuff.Mode mode);
/** * Get a gradient drawable according to the corner radius. * * @param width The width in dip for the drawable. * @param height The height in dip for the drawable. * @param cornerRadius The corner size in dip for the drawable. * * @return The gradient drawable according to the supplied parameters. */@NonNull Drawable getCornerDrawable(int width, int height, float cornerRadius);
DynamicLinkUtils
A collection of functions to perform various operations on the URL
or to generate intents.
/** * View app on Google Play or Android Market. * * @param context The context to retrieve the resources. * @param packageName Application package name to build the search query. * * @throws ActivityNotFoundException If no activity is found. * * @see Intent#ACTION_VIEW */viewInGooglePlay(@NonNull Context context, @NonNull String packageName);
/** * View app on Google Play or Android Market. * Can be used for the quick feedback or rating from the user. * * This method throws {@link ActivityNotFoundException} if there was no activity found * to run the given intent. * * @param context The context to retrieve the resources. * * @throws ActivityNotFoundException If no activity is found. * * @see #viewInGooglePlay(Context, String) */void rateApp(@NonNull Context context);
/** * Checks whether the GMS (Google Mobile Services) package exists on the device. * * @param context The context to get the package manager. * * @return {@code true} if the GMS (Google Mobile Services) package exists on the device. */boolean isGMSExists(@NonNull Context context);
DynamicPackageUtils
Helper class to get package
or app related information.
/** * Checks if a given package name exits. * * @param context The context to get the package manager. * @param packageName The package name to be checked. * * @return {@code true} if the given package name exits. */boolean isPackageExists(@NonNull Context context, @Nullable String packageName);
/** * Get package version name from the given context. * * @param context The context to get the package manager. * * @return The package version name. * * @see android.content.pm.PackageInfo#versionName */@Nullable String getAppVersion(@NonNull Context context);
DynamicUnitUtils
Helper class to perform unit
conversions.
/** * Converts DP into pixels. * * @param dp The value in DP to be converted into pixels. * * @return The converted value in pixels. */int convertDpToPixels(float dp);
/** * Converts pixels into DP. * * @param pixels The value in pixels to be converted into DP. * * @return The converted value in DP. */int convertPixelsToDp(float pixels);
/** * Converts SP into pixels. * * @param sp The value in SP to be converted into pixels. * * @return The converted value in pixels. */float convertSpToPixels(float sp);
/** * Converts pixels into SP. * * @param pixels The value in pixels to be converted into SP. * * @return The converted value in SP. */float convertPixelsToSp(float pixels);
/** * Converts DP into SP. * * @param dp The value in DP to be converted into SP. * * @return The converted value in SP. */float convertDpToSp(float dp);
DynamicSdkUtils
Helper class to detect the Android SDK
version at runtime so that we can provide the user experience accordingly. Pass true
in the alternate method to check for equality.
/** * Detects if the current API version is 14 or above. * * @param equals {@code true} to check for equality. * <p>{@code false} to match greater than or equal. * * @return {@code true} if the current API version is 14 or above. */boolean is14(boolean equals);
/** * Detects if the current API version is 29 or above. * * @return {@code true} if the current API version is 29 or above. */boolean is29();
DynamicViewUtils
Helper class to perform view
operations.
/** * Set hide navigation flag for edge-to-edge content on API 23 and above devices. * * @param view The view to get the system ui flags. * @param edgeToEdge {@code true} to hide the layout navigation. */@TargetApi(Build.VERSION_CODES.M)setEdgeToEdge(@NonNull View view, boolean edgeToEdge);
/** * Set light status bar if we are using light primary color on API 23 and above devices. * * @param view The view to get the system ui flags. * @param light {@code true} to set the light status bar. */@TargetApi(Build.VERSION_CODES.M)void setLightStatusBar(@NonNull View view, boolean light);
DynamicWindowUtils
Helper class to perform window
operations and to detect system configurations at runtime.
/** * Detects support for navigation bar theme. * * @param context The context to retrieve the resources. * * @return {@code true} if navigation bar theme is supported. */boolean isNavigationBarThemeSupported(@NonNull Context context);
/** * Get the current device orientation. * * @param context The context to get the resources and window service. * * @return The current activity orientation info. * * @see ActivityInfo#SCREEN_ORIENTATION_PORTRAIT * @see ActivityInfo#SCREEN_ORIENTATION_REVERSE_PORTRAIT * @see ActivityInfo#SCREEN_ORIENTATION_LANDSCAPE * @see ActivityInfo#SCREEN_ORIENTATION_REVERSE_LANDSCAPE */int getScreenOrientation(@NonNull Context context);
Installation
Please follow the GitHub repository for the installation guide. I hope it will help you in your projects and please ask questions or share your feedback in the comments section to make it better.